Android automation testing is a must for most applications. Why? Because, to put simply, it ensures that functionality is consistent across different devices and Operating Systems (OS) versions. In this article, we explain step by step how one can implement Android automation testing on popular tools and frameworks, which saves time and gets more accurate …
Android Automation Testing: A Step-by-Step Tutorial
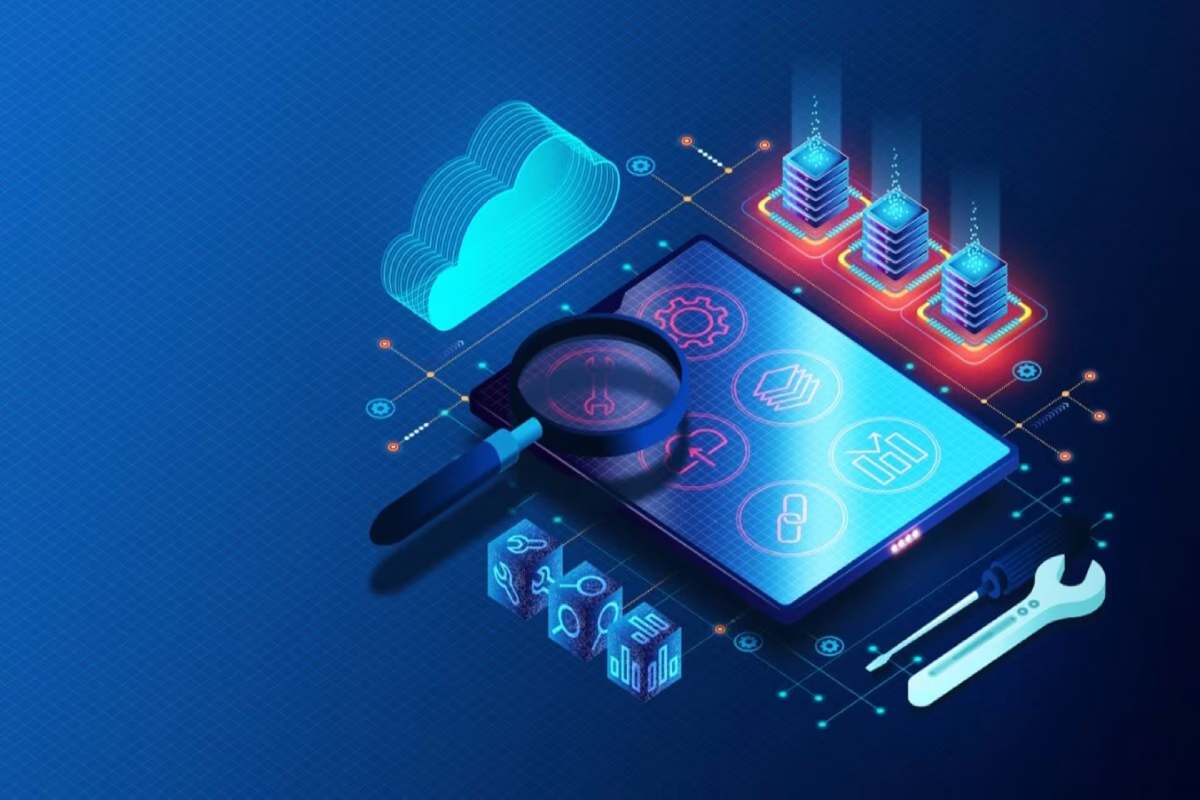
Android automation testing is a must for most applications. Why? Because, to put simply, it ensures that functionality is consistent across different devices and Operating Systems (OS) versions.
In this article, we explain step by step how one can implement Android automation testing on popular tools and frameworks, which saves time and gets more accurate results than manual testing.
Let’s begin!
What is Android Automation Testing?
Android automation testing is the process of testing Android applications through software scripts. This technique enables testers to simulate various user actions, such as tapping buttons or entering text, on multiple devices at a time. By this, organizations can ensure that their applications perform well under different conditions and save time spent in manual testing.
Why Use Automation Testing?
The mobile app market is expanding all the time, and making sure your apps run well on any given device is essential. Testing with a lot of different devices would be labor-intensive; the automation testing process ensures that an organization tests many more scenarios faster.
Moreover, it is essential to ensure that the test team conducts tests continuously in this fast-paced development environment.
Benefits of Android Automation Testing
The following are the benefits of Android automation testing:
- Speed
Automated tests can run much faster than manual tests, so organizations should prefer automated tests.
- Reusability
Once you have written test scripts, you can reuse test scripts from one version of the application to another.
- Consistency
Automated tests avoid all human error, meaning consistency in results every time tests are run.
- Scalability
Automation helps scale up your testing because one can run tests on different devices at the same time.
- Early Bug Detection
Automated tests can easily be incorporated into the development process as they start catching bugs when they just reach the early stages of production.
- Cost-Effective
It is cost-effective, though one could incur a bit of expense to set it up, though the reduced efforts are enormous in comparison.
- Huge Test Case Coverage
Automation allows the team to cover more test cases than practical to do them in the time required for conducting manual tests.
Setting Up the Environment
To start writing tests for Android, one needs to install the following:
It is the official Integrated Development Environment (IDE) for Android that provides all the things required to make, test, and polish Android applications.
Step 1: Installation of Android SDK
This comprises libraries and tools for building and testing Android applications. Install Android Debug Bridge. This allows for communication between your computer and Android devices.
Step 2: Open Android
Go to Android Device Manager, create a new virtual device, and then start the emulator for testing on an emulator or physical device.
Step 3: Install dependencies or libraries.
Install Node.js for Appium and build Gradle for Espresso according to the framework chosen.
Step 4: Set Up Your Project Structure
Make a separate directory for tests within your project. Keep them organized by functionality or feature. Use naming conventions that indicate each test’s function.
In short, these steps are required to create and maintain successful test scripts for Android development.
Choosing an Automation Framework
Many frameworks are available for Android automation testing. Some of the most popular ones include the following:
- Appium
The open-source tool that supports several programming languages and allows a cross-platform test of native, hybrid, and mobile web applications is known as Appium.
It is widely used in mobile automation due to its flexibility and compatibility with various platforms. Key features include support for Java, Python, Ruby, Selenium WebDriver, and real devices and emulators/simulators.
- Espresso
Google’s Espresso is the testing tool for Android UI, well-integrated with Android Studio, and provides several APIs for interacting with User Interface (UI) components. Its main features are built-in synchronization, easy integration with projects already existing on Android, a fluent API for creating concise test scripts, and strong assertions for validation of UI state.
- Robotium
Robotium is a robust tool for native and hybrid app testing with user-friendly APIs for UI interaction. It makes the automation of test writing very simple, hence ideal for developers who wish to write tests quickly without much setup. Key features include support for advanced user interactions like gestures and integration with other frameworks like JUnit.
- Selendroid
Selendroid is an open-source framework that allows testing native and hybrid Android applications on older devices. It supports both emulators and actual devices, performs well with Selenium WebDriver, and also allows element inspection through a built-in inspector tool. This makes it suitable for legacy system support.
- Detox
Detox is a powerful testing framework tailored to react to native applications. Thus, it gives an excellent choice for cross-platform app development. It provides full support, synchronization with animations and network requests, has a powerful Command-Line Interface (CLI) tool for testing, and supports scenarios from unit level to end-to-end.
Writing Your First Test
Once you have selected your framework, it is time to write your first automated test script. The following steps guide how to create a simple test case using Appium as an example.
Step 1: Set Up Appium
To install Appium, download it from the official website or use Node.js via npm. Start the server using the command line or Appium Desktop.
Step 2: Create a Test Script
A test script is a process that defines desired capabilities and instructions for a test case, such as device information, application package name, and other Appium-specific settings. For instance, a test script may comprise launching the app, entering the username and password, clicking the login button, and verifying the home screen’s appearance.
Step 3: Run Your Test
After writing your test script, run it from your command line or preferred IDE while ensuring that your emulator or physical device is connected and ready for testing.
Best Practices in Android Automation Testing
To maximize the effectiveness of your Android automation tests, consider these best practices:
- Understand Your Application
Before writing test cases, it’s essential to understand the application’s functionality and user flows. This knowledge helps testers create relevant tests that cover all paths in the application.
Mapping user interactions can identify critical scenarios that require exhaustive testing, ensuring tests are more similar to actual user behavior. Engaging with stakeholders like product managers and developers can enhance test coverage and identify pitfalls.
- Use Version Control
Version control systems like Git are essential for tracking changes in test scripts, enabling the team to identify issues and revert to previous versions. Branching strategies enable multiple developers to work on different features simultaneously without conflicts. Regular code reviews help identify problems early and improve the quality of test code.
- Run Tests Frequently
Automation tests integrated with the Continuous Integration/Continuous Deployment (CI/CD) pipeline can detect issues early in development, maintain high code quality, and be scheduled at off-peak hours for consistent execution. Monitoring results from CI/CD pipelines can identify failure trends or flaky tests, providing valuable insights into areas needing extra attention.
- Keep Your Tests
Regularly reviewing and updating test cases is crucial for the application’s evolution. Old tests should be discarded, and new ones should be introduced as new features are added.
This refactoring of complex test code enhances maintainability and reduces error-prone occurrences. Automating maintenance tasks, such as cleaning old test cases and updating dependencies, allows teams to focus on other critical testing activities.
- Prioritize Test Cases
Not all test cases are the same, and so priority must be given to critical user flows and high-risk areas of the application. The idea here is to concentrate on those scenarios that most affect user experience or organizational objectives, thus directing resources where they have maximum value.
Risk-based testing strategies thus help find those areas that are more prone to failure or have higher impacts on users and thereby doing direct tests at places that matter.
- Implement Reporting Mechanisms
Reporting tools can be incorporated into your automation framework such that detailed reports are generated after each test run. These should include pass/fail ratios, execution times, and even errors encountered, thus making them easy to understand for your stakeholders.
Custom dashboards highlighting key metrics can further improve visibility around testing outcomes. Feedback loops need to be established where stakeholders analyze results and provide input to continuously improve processes around testing.
- Invest in Training
Investing in team members’ skills is crucial for maintaining high standards in automation testing. As technology evolves, well-versed testers stay sharp. Knowledge sharing within the team fosters a collaborative environment, with experienced members mentoring others, sharing resources, and conducting workshops on effective automation strategies.
This commitment to continuous learning leads to improved testing outcomes and a more proficient team.
- Use Automation Frameworks Wisely
Select the most appropriate automation framework that matches the needs of your project for optimum testing efficiency. Standardizing scripting practices ensures uniformity in test scripts, thus making them more readable and maintainable.
Design patterns such as the Page Object Model (POM) encapsulate UI elements and interactions, thus making the script more resistant to changes in the user interface.
Leveraging data-driven testing approaches allows teams to run the same tests with different inputs, increasing coverage without duplicating effort, while consistently monitoring key metrics helps assess the impact of automation accurately.
By following these best practices in Android automation testing, teams can considerably enhance their effectiveness and efficiency in delivering high-quality applications that meet user expectations consistently.
- Cloud Testing
As organizations scale their applications, cloud-based solutions become increasingly valuable. Cloud testing platforms such as LambdaTest provide many advantages for Android automation testing.
LambdaTest is an all-inclusive automation testing platform offering access to more than 3000 real desktop and mobile environments, allowing complete compatibility testing across devices.
Parallel testing is also possible through this tool, thereby reducing testing time and enabling faster updates without affecting quality. LambdaTest also provides auto-healing for maintaining test reliability, wherein the tool automatically recovers from failures without human intervention.
The platform is SOC 2 Type 2 certified and GDPR compliant, ensuring data security while undergoing automated testing processes. With LambdaTest, one instantly gets access to more than 3000 real browsers and devices through its cloud platform, which gives one the ability to check comprehensive cross-browser compatibility without extensive local setups or configurations.
For those using an Android emulator Mac, LambdaTest enhances automation efforts by offering a reliable infrastructure for running tests at scale while making sure that it is also in compliance with industry standards. This makes it an excellent choice for teams looking to streamline their testing processes and improve overall application quality.
- CI/CD
Integrating automated tests into CI/CD pipelines improves efficiency by running tests automatically when code changes occur. Tools like Jenkins or CircleCI can automate the process further. Benefits include faster feedback, reduced manual effort, and improved collaboration.
To implement CI/CD pipelines, select a tool such as Jenkins, configure build jobs with automated tests after each build, and set up notifications for failed builds to alert developers proactively. This ensures quality control throughout development cycles and improves communication between teams.
- Performance Testing
Functional testing is essential for ensuring that an application functions well under load conditions. Tools such as JMeter and Gatling can be included in automation strategies to measure critical performance metrics such as response time, throughput, and resource utilization.
Performance tests define performance criteria based on the expectations of the users, create performance scripts simulating real user behavior under load conditions, and then analyze results against defined benchmarks to identify bottlenecks and make informed decisions on optimizations.
- Cross-Platform Testing
Cross-platform development using Appium for apps on both Android and iOS ensures consistency, saves costs, and has a quicker time to market. This minimizes duplication of effort, saves time and resources, and enables simultaneous updates across platforms to provide a seamless user experience, regardless of the device used.
Conclusion
To conclude, Android automation testing enhances development efficiency by delivering high-quality applications on various devices. Frameworks like Appium, Espresso, Robot Framework, and platforms like LambdaTest enable quick adaptation to market demands. This meets user expectations and provides a competitive advantage in the fast-paced mobile application landscape, allowing developers to sharpen their skills and accomplish more efficiently.